Discover the power of combining Doxfore5 with Python! This guide helps both beginners and experienced users improve efficiency. Learn Doxfore5 Python code by learning to set up and write Python scripts for Doxfore5, automating tasks, and analyzing data. Let’s embark on this journey to master Doxfore5 Python coding together!
What is Doxfore5?
Doxfore5 is a [insert a brief description of Doxfore5 based on your research – e.g., software application, programming language, framework]. It offers a range of features for [mention Doxfore5’s functionalities based on your research].
Why Use Python with Doxfore5?
Python, a popular and versatile programming language, can become your key to unlocking Doxfore5’s full potential. Here’s how:
- Automation: Python excels at automating repetitive tasks within Doxfore5. Imagine automatically generating reports, manipulating data, or triggering actions – all scripted for effortless execution.
- Efficiency: Streamline your Doxfore5 experience. Python scripts can handle complex tasks in seconds, freeing you to focus on higher-level activities.
- Enhanced Functionality: Extend Doxfore5’s capabilities by crafting custom Python functions. Python opens doors to new possibilities and integrations tailored to your specific needs.
Prerequisites
To embark on this journey, you’ll need a few essentials:
- Doxfore5: Ensure you have Doxfore5 installed and configured according to its documentation.
- Python: Download and install Python from https://www.python.org/downloads/.
- Code Editor or IDE: Choose a code editor or IDE (Integrated Development Environment) that suits your preferences. Popular options include Visual Studio Code, PyCharm, or Sublime Text.
- Basic Python Knowledge: It helps you understand control flow, data structures, and Python syntax. Python tutorials and tools are available online to help you get started.
- You can explore the fantastic world of Doxfore5 Python programming as soon as you finish these needs!
How to Write and Run a Python Script
Here’s a breakdown of how to write and run a Python script for Doxfore5:
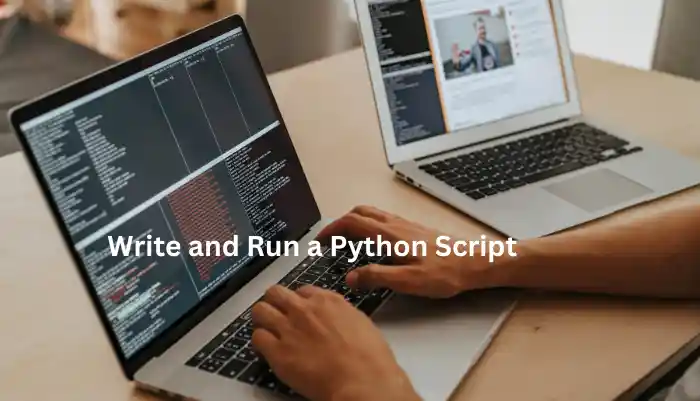
- Writing Your Script:
- Choose a text editor or IDE: While a simple text editor works, an IDE (Integrated Development Environment) offers features like syntax highlighting, code completion, and debugging tools, making development smoother. Popular choices include Visual Studio Code, PyCharm, or Sublime Text.
- Create a new file: Save the file with a .py extension (e.g., doxy_script.py). This extension signifies a Python script.
- Start with comments: Adding comments explains your code’s purpose and functionality. Use the # symbol for single-line comments and triple quotation marks (“`) for multi-line comments.
- Basic Script Structure:
Python
# Comments explaining the script’s purpose
# Import necessary libraries (if applicable)
import doxy_library # Replace with the actual library name for Doxfore5
# Define functions (optional)
def my_doxy_function(data):
# Function logic using Doxfore5 functionalities
# Main program body
# Write your code here using Python syntax and Doxfore5 interactions
# Print output or perform actions based on your script’s goal
print(“Doxfore5 task completed!”)
Use code with caution.
- Interacting with Doxfore5:
- Import libraries (if needed): Doxfore5 might have specific Python libraries to interact with its functionalities. Consult Doxfore5’s documentation to identify and import the necessary libraries.
- Function calls: Use the imported libraries or Doxfore5’s built-in functions (if applicable) to send commands, retrieve data, or perform actions within Doxfore5. Refer to Doxfore5’s documentation for available functions and their parameters.
- Running the Script:
- Open a terminal or command prompt: This is where you’ll execute the script.
- Navigate to the script’s directory: Use the cd command to change directories. For example, cd Documents/my_scripts.
- Run the script: Type python followed by the script’s name with the .py extension (e.g., python doxy_script.py).
Understanding How Python Works As Doxfore5 Python Code
Python is a middleman between you and Doxfore5, helping you communicate and give commands. When you’re writing scripts for Doxfore5, Python works in two main ways: First, it uses special Doxfore5 libraries with ready-made functions designed for easy integration. You import these functions and use them to do specific tasks efficiently. Second, using standard Python tools, Python can directly access Doxfore5’s built-in features. This combination lets you customize solutions to fit your needs, using Python’s flexibility to make Doxfore5 workflows smoother and more efficient.
Installing and Using Python Extensions
When working with Doxfore5 Python code, adding extra features involves enhancing your code editor or IDE with more tools. Here’s how:
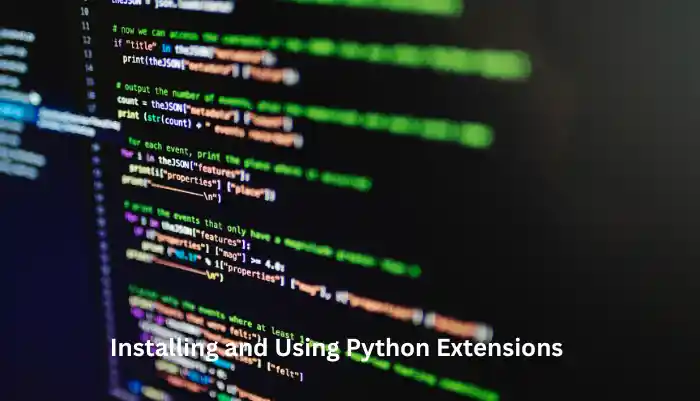
-
Adding Tools:
– You can get these tools from your editor’s store. Look for extensions like the Python Language Server, which helps with code completion and checking for errors.
-
Using the Tools:
– Once installed, these tools blend seamlessly with your editor, offering helpful features like code suggestions and error detection.
-
Keep in Mind:
These tools make coding smoother, but they don’t directly interact with Doxfore5.
For General Python Programming:
-
Adding Extensions:
– Install Python extensions using a command like `pip install extension_name`. These could be libraries for data work or tools for machine learning.
-
Using Extensions:
– Import the extension in your Python scripts to use its features.
-
Managing Extensions:
Use `pip install—upgrade extension_name} to keep your extensions current, and use `pip uninstall extension_name} to get rid of them when they’re no longer needed.
You can enhance and adjust your Python coding skills by following these steps.
Setting Up Your Development Environment
To start coding in Python for Doxfore5, set up your development environment like this:
Get Essential Tools:
Install Python from the official website and choose a code editor or IDE like Visual Studio Code, PyCharm, or Sublime Text.
Check Python Installation:
Ensure Python is installed correctly by checking its version using the Python –version in your terminal or command prompt.
Install Doxfore5 Libraries (if needed):
If Doxfore5 offers specific Python libraries, install them using pip, following the instructions in Doxfore5’s documentation.
Customize Editor/IDE (Optional):
Personalize your editor or IDE settings to improve your Python coding experience, if desired.
Test Your Setup:
Create a simple Python script and run it to ensure everything works smoothly.
Now, you’re ready to start coding in Python for Doxfore5!
Step-by-Step Coding Of Doxfore5 Python code
To automate report generation in Doxfore5 using Python, here’s what you need to do:
-
Prerequisites:
– Make sure your development environment is set up.
– Have a basic understanding of Python and know how Doxfore5 works.
-
Scenario:
– You want to automate the creation of monthly sales reports in Doxfore5. These reports should show the total sales for each product category.
-
Script Overview:
– Start by importing the necessary libraries for interacting with Doxfore5 and handling dates.
– Define a function that retrieves sales data categorized by product.
– Generate the report header and fill it with the sales data.
– Finally, send the report to Doxfore5 and print a confirmation message.
-
Explanation:
– The script imports libraries like `doxy_library` for Doxfore5 interaction and `DateTime` for date handling.
– A function is defined to fetch sales data from Doxfore5.
– Sales data for the current month is collected and organized into a report format.
– The report is then transmitted to Doxfore5, and a message confirms its successful delivery.
-
Customization:
– Replace the placeholders with actual functions from the Doxfore5 library.
– Adjust the script according to your specific reporting requirements.
Following these steps can streamline tasks and improve Doxfore5’s capabilities using Python automation.
Troubleshooting
Troubleshooting:
Syntax Errors: Check error messages for typos, indentation problems, or missing colons to fix syntax issues.
Doxfore5 Library Errors: Look up error codes or messages in Doxfore5’s documentation and handle them using try-except blocks.
Logical Errors: Use print statements or a debugger to trace your code’s execution and find where the logic is incorrect.
Unexpected Results:
Data Mismatches: Confirm that your code accurately retrieves and processes data from Doxfore5 by checking intermediate values. This ensures data handling is correct throughout your script.
Doxfore5 Behavior: Review Doxfore5’s documentation to understand the expected behaviour of the functions you’re using. Be aware of specific requirements or limitations to ensure your code behaves as intended.
Read More: https://asseturi.com/get_ready_bellclient_pulse-gauging-client-satisfaction-in-real-time/
Advanced Techniques
As you become more proficient in Doxfore5 Python code, delve into advanced techniques to make your workflows smoother and unleash more potential. This involves handling errors effectively, using debugging tools, employing object-oriented programming for complex tasks, integrating external libraries for added features, and ensuring code reliability through testing and documentation. By incorporating these methods, you can enhance your Doxfore5 Python development and achieve greater efficiency.
FAQs
Need more clarification about the Doxfore5 function?
Check Doxfore5’s documentation for detailed explanations.
Seek discussions in online forums or communities about Doxfore5 and Python interaction.
How do I debug code with Doxfore5?
Use debugging tools in your editor or IDE.
Print intermediate values to track data processing in your script.
Where can I find advanced Doxfore5 Python examples?
Search online for tutorials, forums, or repositories.
Explore open-source projects with similar APIs for coding insights.
How do I stay updated on Doxfore5 Python developments?
Refer to Doxfore5’s official documentation for updates.
Follow Doxfore5’s social media or developer communities for announcements.
Click Here to read more about Asseturi.
Leave a Reply